字符串的一些关键点
整数与罗马数字的转换
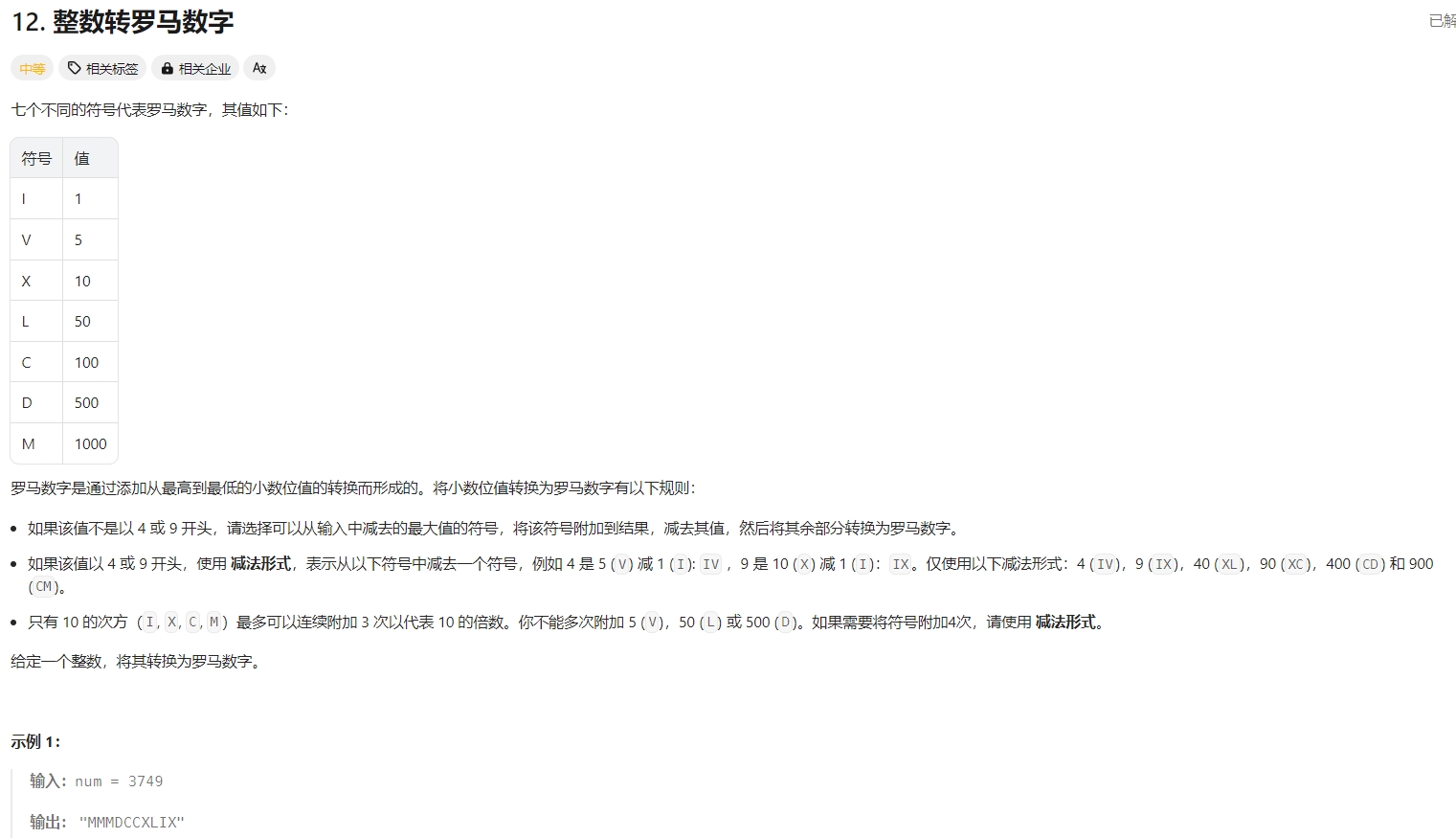
Click to expand the code
class Solution {
public:
// 通过vector穷举了所有的可能性(由于不同的千百十位对应不同的字符,故此穷举最直接)
vector<pair<int, string>> group = {
{1000, "M"},
{900, "CM"},
{500, "D"},
{400, "CD"},
{100, "C"},
{90, "XC"},
{50, "L"},
{40, "XL"},
{10, "X"},
{9, "IX"},
{5, "V"},
{4, "IV"},
{1, "I"},
};//顺序很重要!
string intToRoman(int num) {
string output_str;
// 遍历这个group
for(int i=0;i<group.size();i++)//长度固定因此复杂度为O(1)
{
// 注意遍历是从大到小减的~
while(num>=group[i].first)//通过while循环,取完了,i再变
{
output_str+=group[i].second;
num=num-group[i].first;
}
if(num==0)
break;
}
return output_str;
}
};
穷举的时候,用map也可以,但是map默认是按key从小到大排列了的,改一下即可~
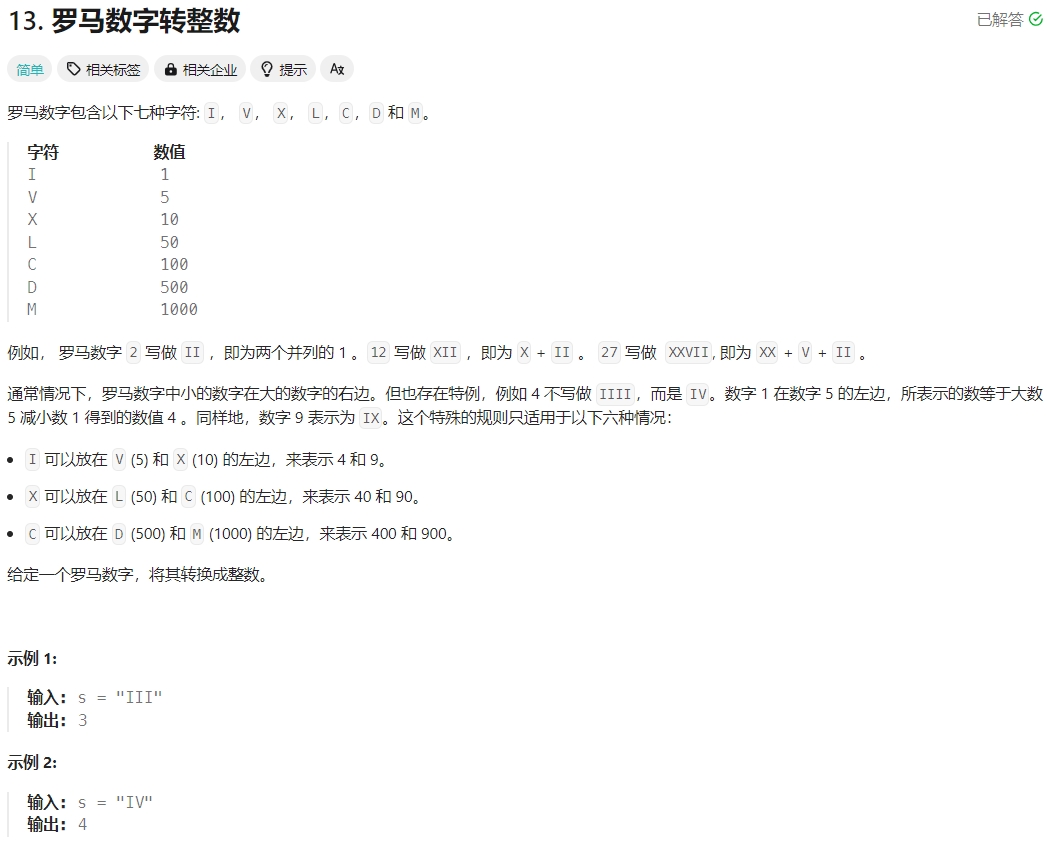
跟上面很类似,只是反过来由罗马变为阿拉伯数字而已~
class Solution {
public:
unordered_map<char, int> define_group=//注意是从小到大
{
{'I',1},
{'V',5},
{'X',10},
{'L',50},
{'C',100},
{'D',500},
{'M',1000}
};
int romanToInt(string s) {
int num_value=0;
for(int i=0;i<s.size();i++)
{
// 若字符串的当前位对应的数字小于下一位对应的数字(对应特殊的4,9等等)
if(define_group[s[i]]<define_group[s[i+1]])
{
//逐个遍历,逐个相加即可~
num_value+=define_group[s[i+1]]-define_group[s[i]];
i=i+1;//当前位和下一位都算了,可以跳过
}
else//反之,则是当前位和下一位相同
num_value+=define_group[s[i]];//直接获取值
}
// num_value=num_value+define_group[s[s.size()-1]];//最后一个加上
return num_value;
}
};
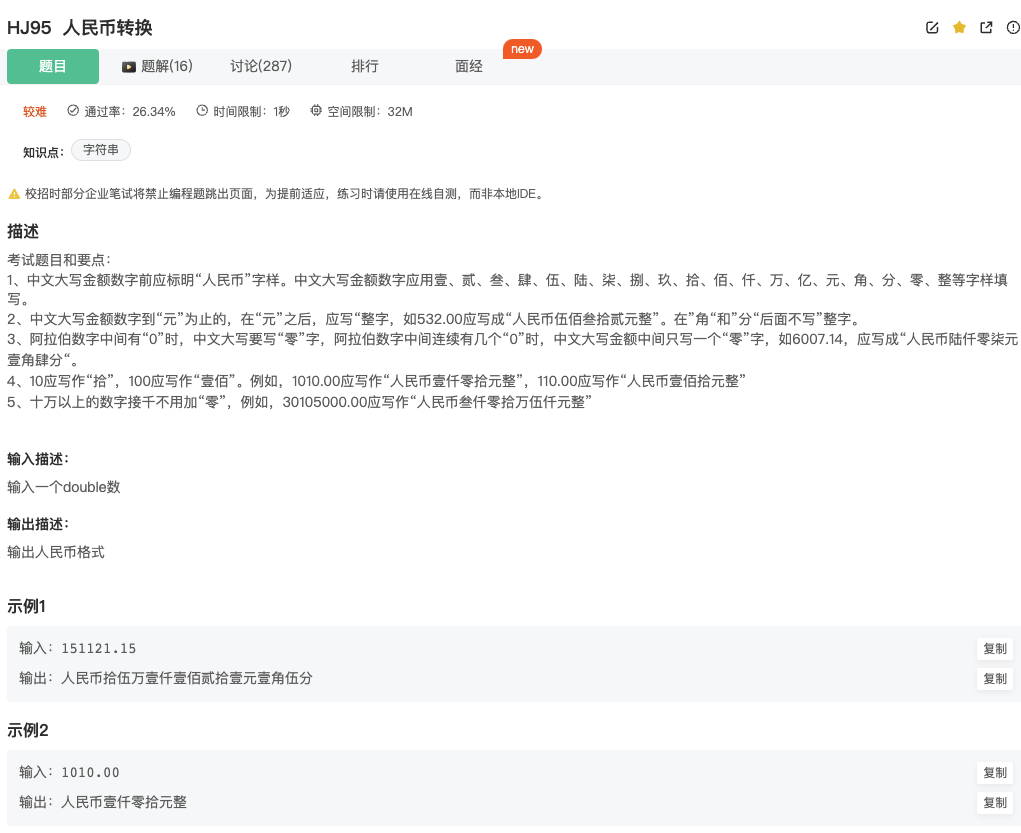
人民币的转换比罗马数字难多了,主要是还要考虑不同位的念法不一样。此处单纯给出代码,还没理思路~
进制转换
进制转换是非常经典的题目,其实关键点都是十进制与其他进制相互转换而已~
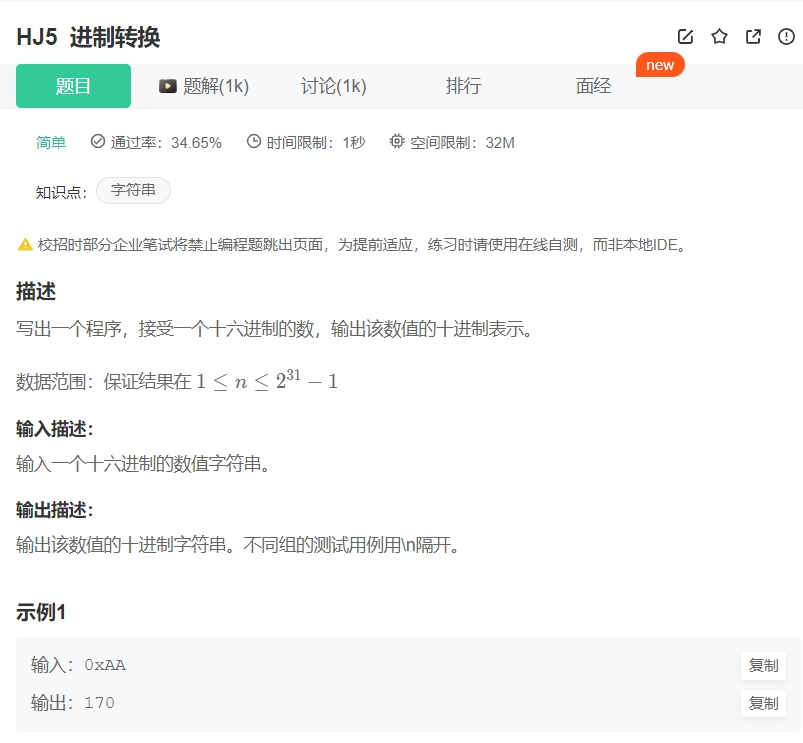
Click to expand the code。注意16进制就是要乘16次幂(pow(16,n))。输入的为字符串,每次对比是字符'0'和'9'
二进制求和
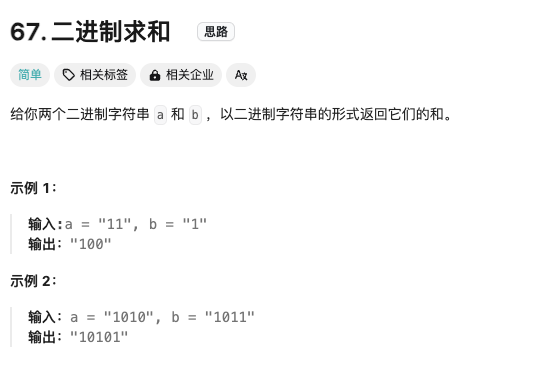
要用string来解题才可以,如果先转换为十进制然后相加可能出现越界情况.时间复杂度, O(Max(M,N))
在内存中存储时1个数
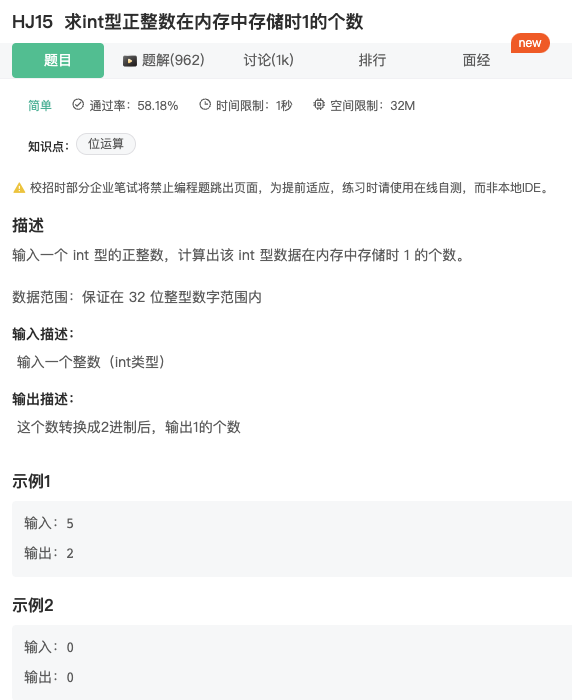
此题实际上就是转换为二进制
求最大连续bit数
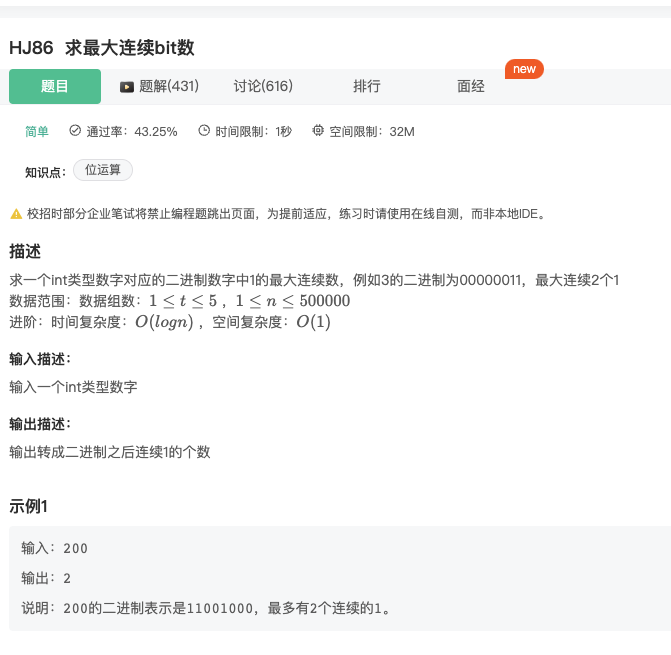
跟上一题是很像的,主要是要注意设计循环迭代求最值都需要对比一下最后一个
整数与ip地址之间的转换
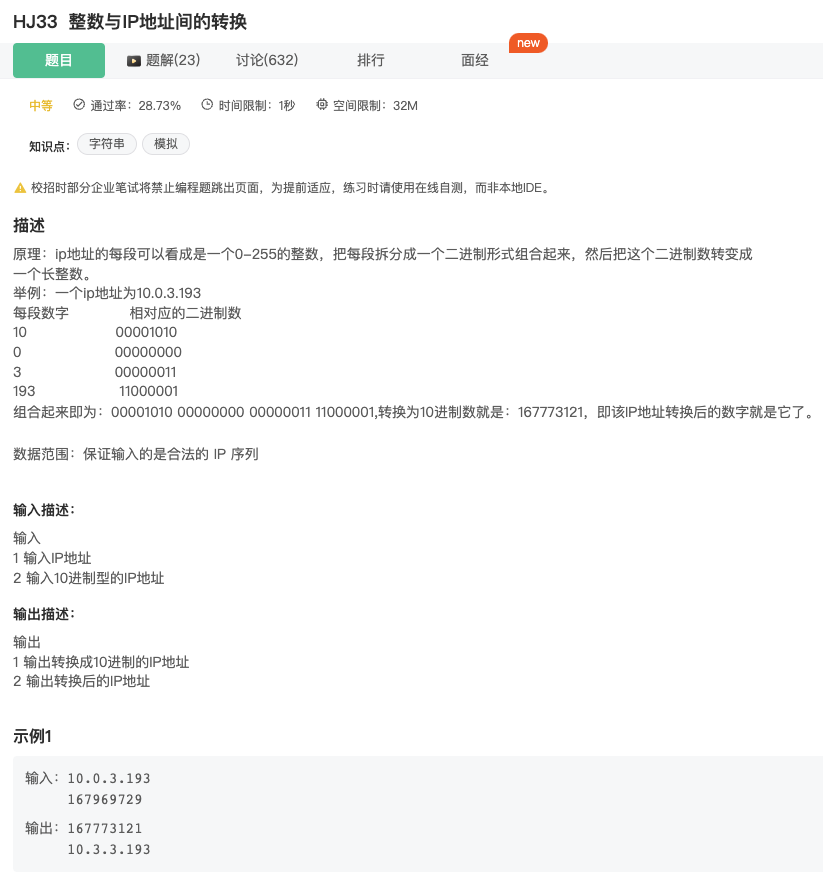
此题包含了十进制转2进制;2进制转10进制。注意n进制转10进制都是pow(n,第几位)逢n进位,故此满一位就是要乘n。细节的地方主要是分组+0的讨论。
Hash Table相关题目
unordered_map内部的元素是无序的,即插入的顺序和输出的顺序不一定相同。而map内部的元素是有序的,它是按照元素的键值大小进行排序,所以它的内部元素是有序的。
定义
合并表记录
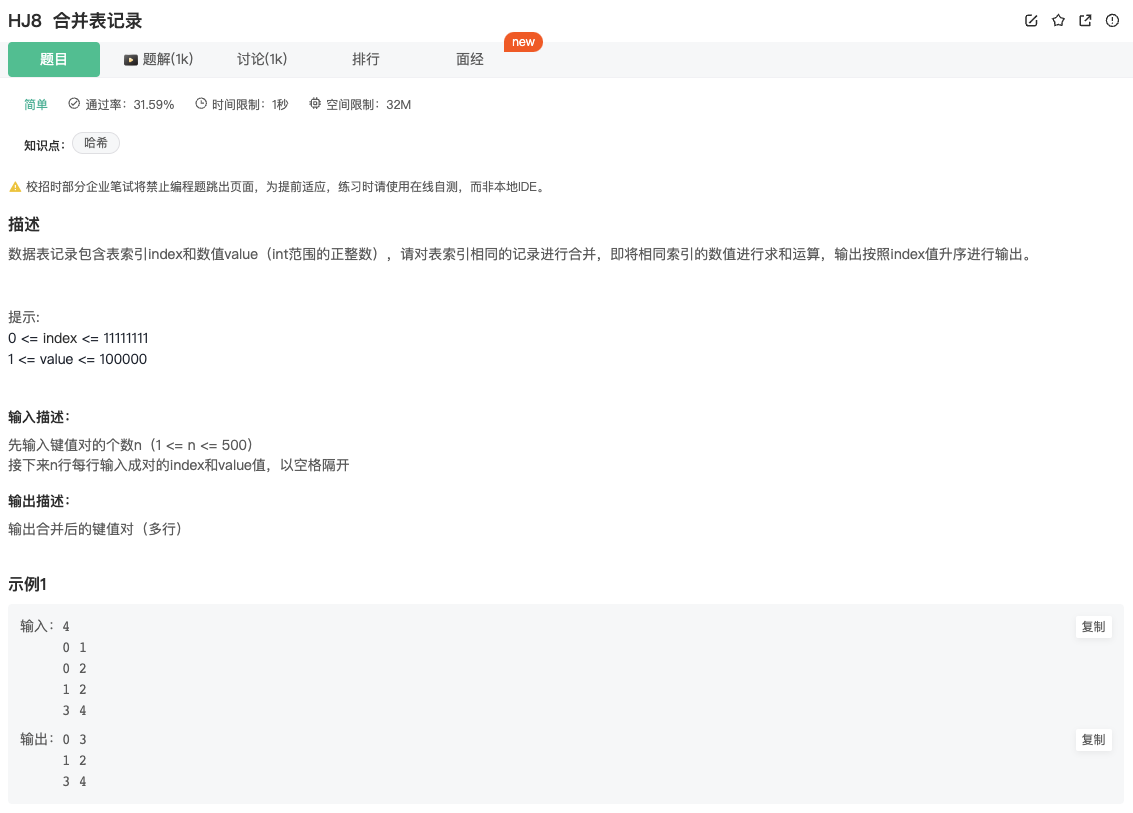
Click to expand the code
简单密码
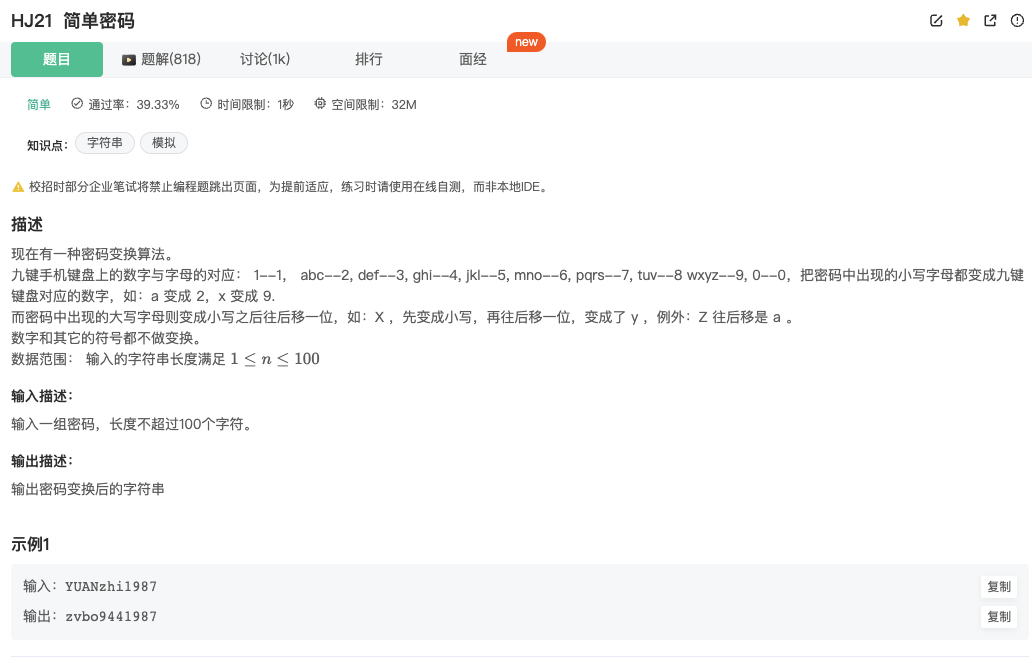
Click to expand the code。关键在于hash table的初始化
称砝码
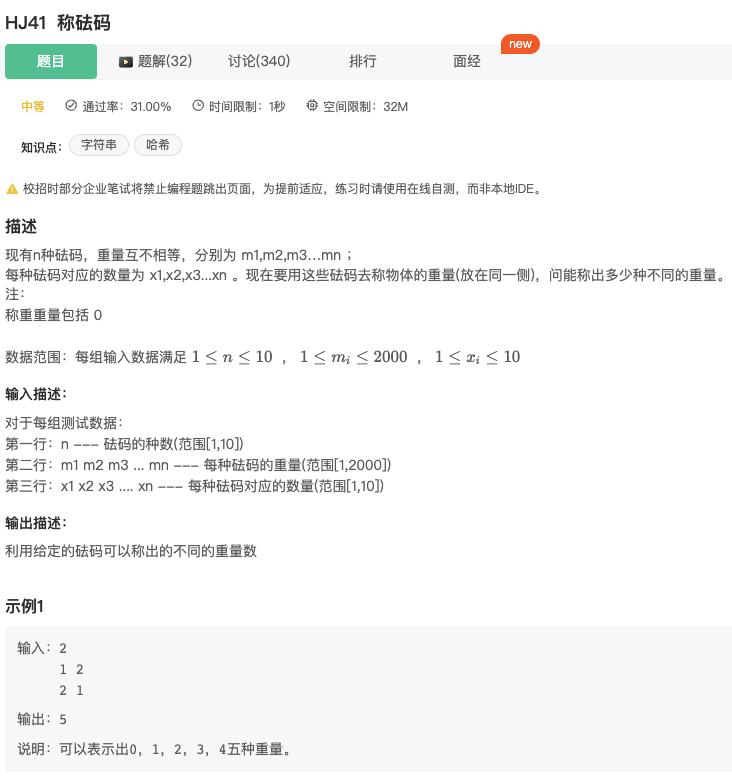
一开始是想着用回溯算法去解的,但是复杂度比较高,且剪枝策略不正确,改为hash table来算吧~
hash table的思维,vector的解法:名字的漂亮度
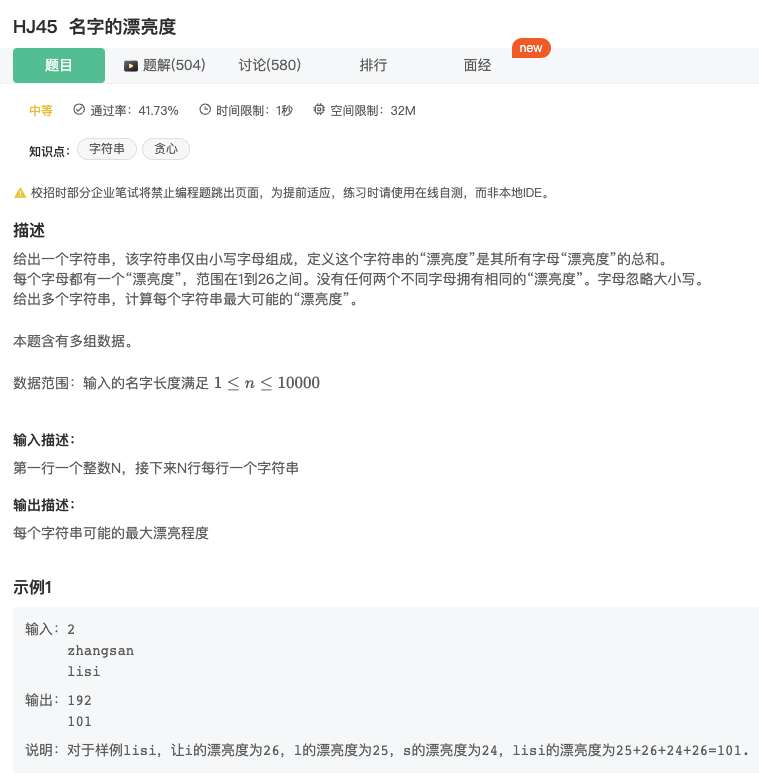
此题是hash table的思维,但是hash table不好进行排序,因此用vector代替,对应位置each_string[each_char-'a']填入即可实现计数
set与map自动升值排列:整型数组合并
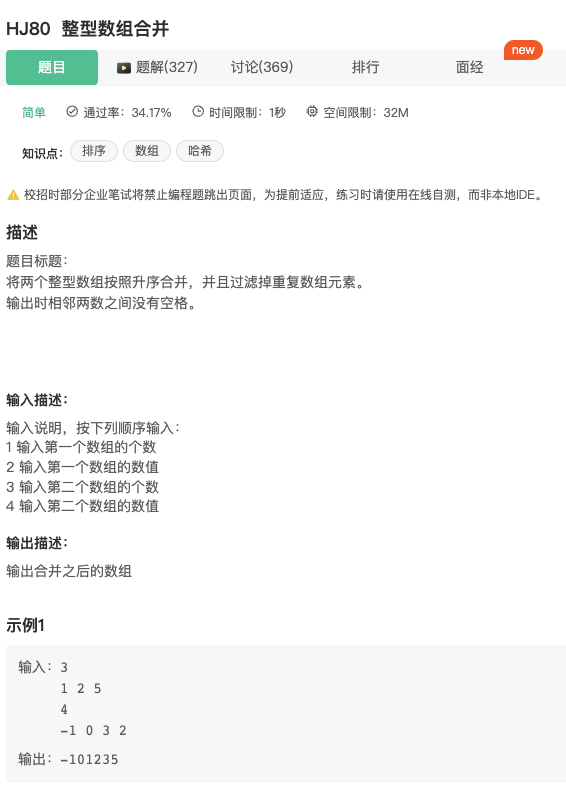
Click to expand the code.两种解法均可~
set通过.count(each_str)来代替find寻值:字符串字符匹配
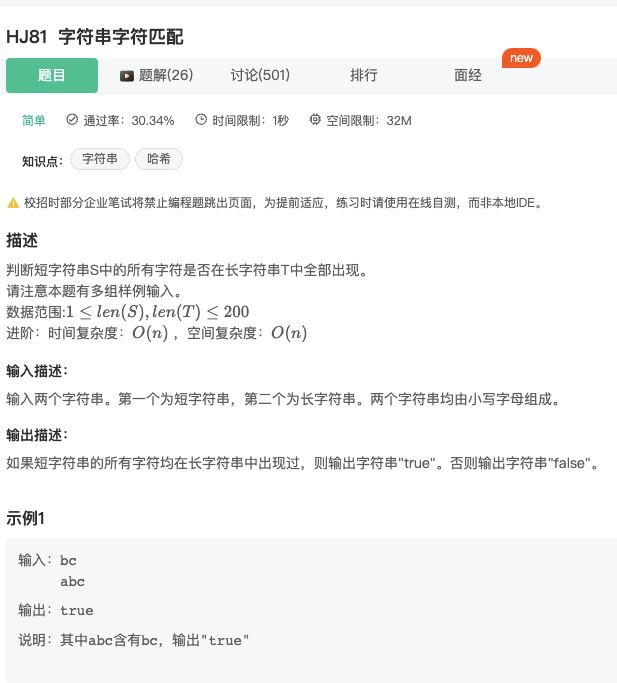
Click to expand the code
Click to expand the code,第二种解法其实就是换个思路,感觉差不多~
将map转换为vector进行排序:字符统计
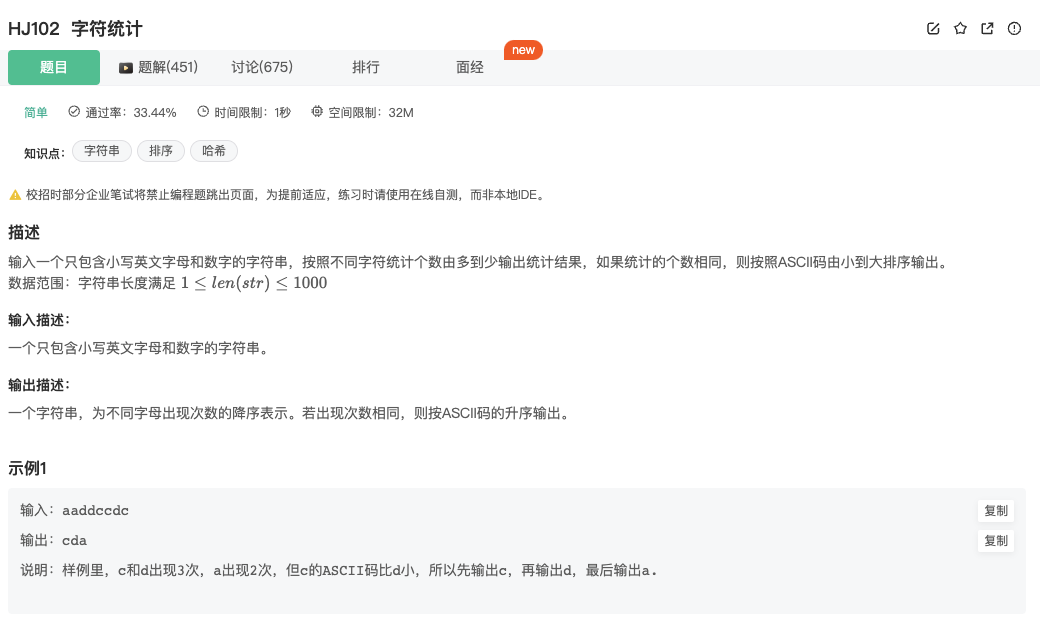
Click to expand the code
其他string相关的题目
字符串最后一个单词的长度
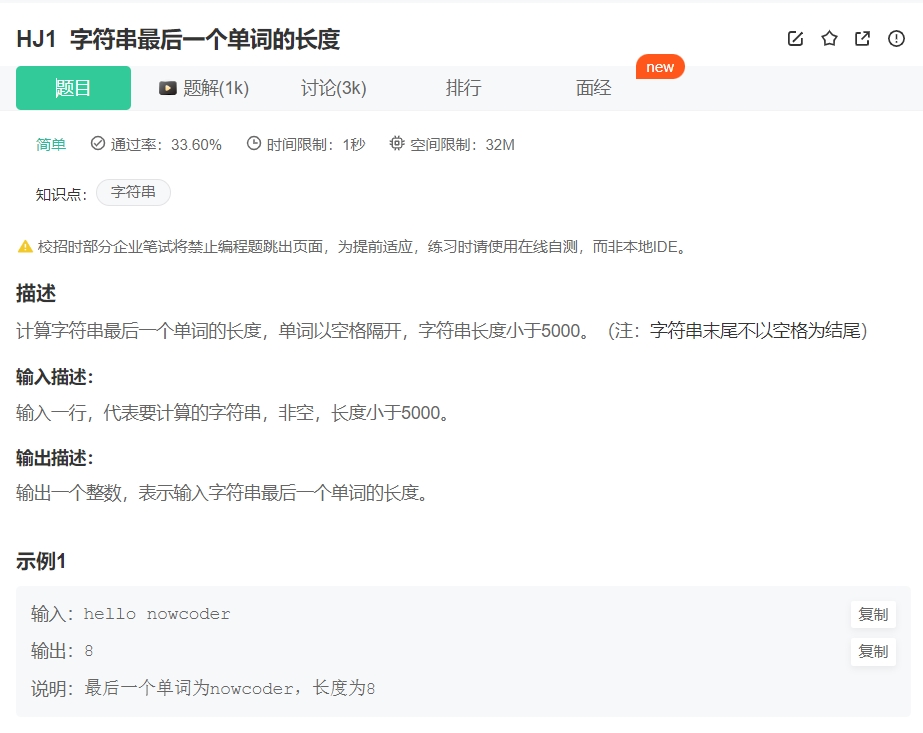
Click to expand the code,trick:输入为空格的时候就停止输入
计算某字符出现次数
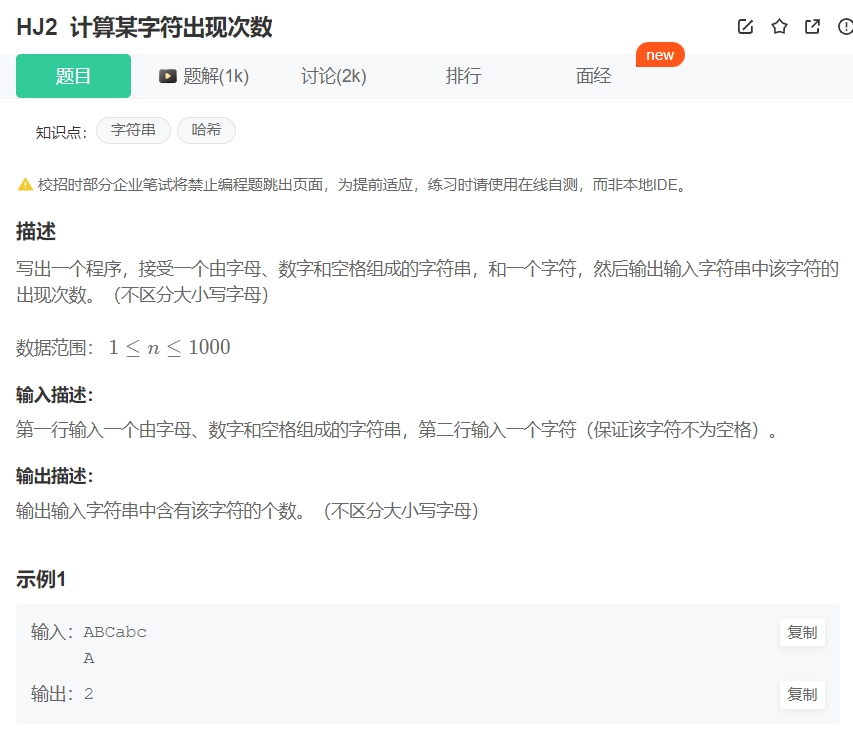
注意不区分大小写,需要调用tolower()函数转换成小写。时间复杂度为O(N),空间复杂度为O(1)
下面方法采用hash table,也可以,但是空间复杂度应该是O(N),时间复杂度一样
明明的随机数
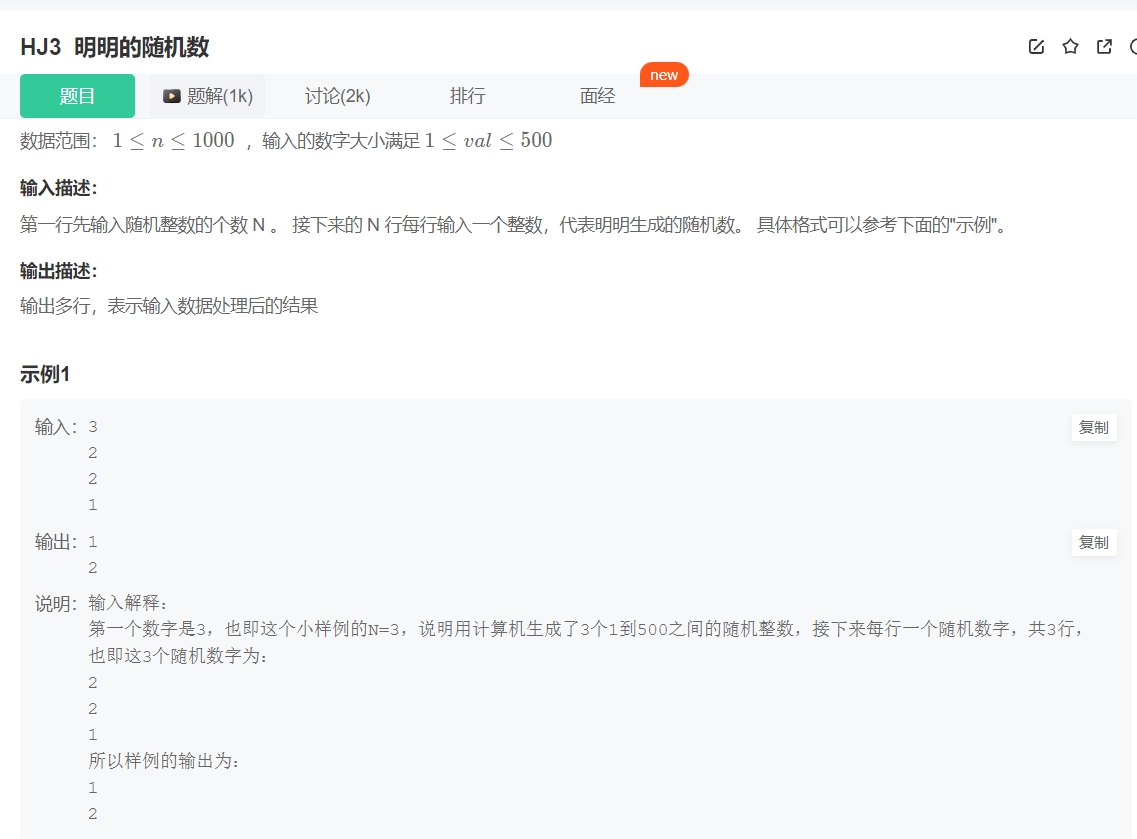
题目:明明生成了N个1到500之间的随机整数。请你删去其中重复的数字,即相同的数字只保留一个,把其余相同的数去掉,然后再把这些数从小到大排序,按照排好的顺序输出。
Click to expand the code。此题的关键是set的使用
字符串分隔
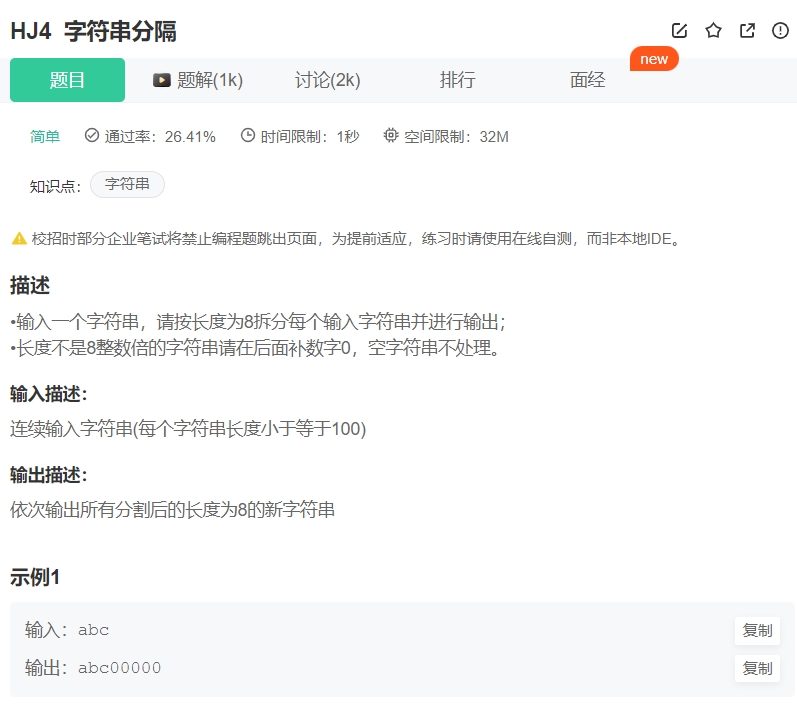
Click to expand the code
提取不重复的整数
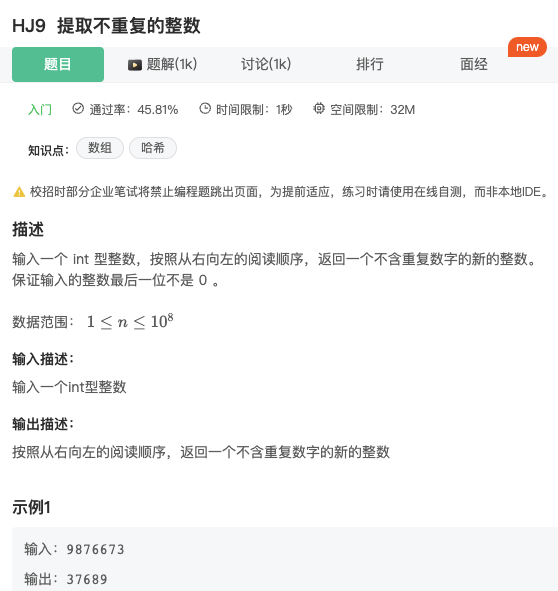
Click to expand the code
string颠倒:数字颠倒
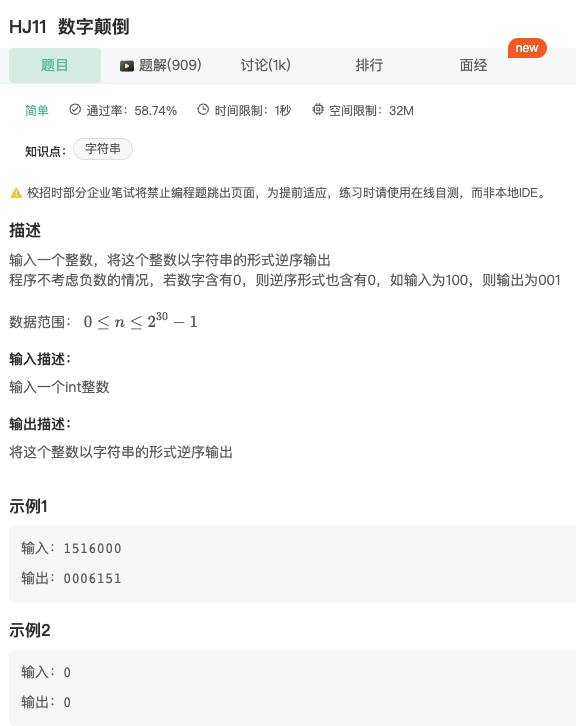
Click to expand the code
字符串排序
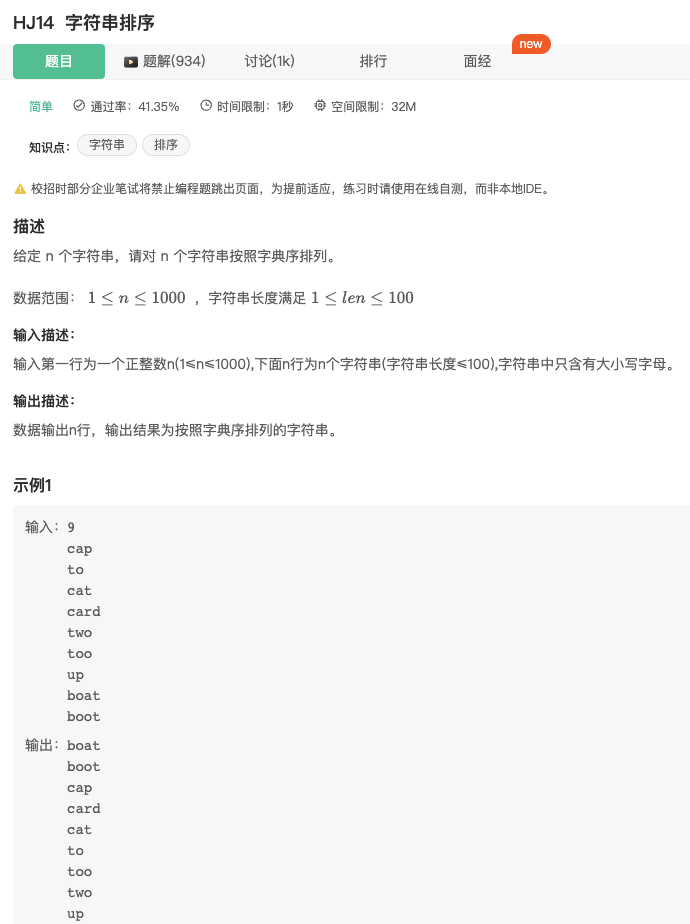
Click to expand the code
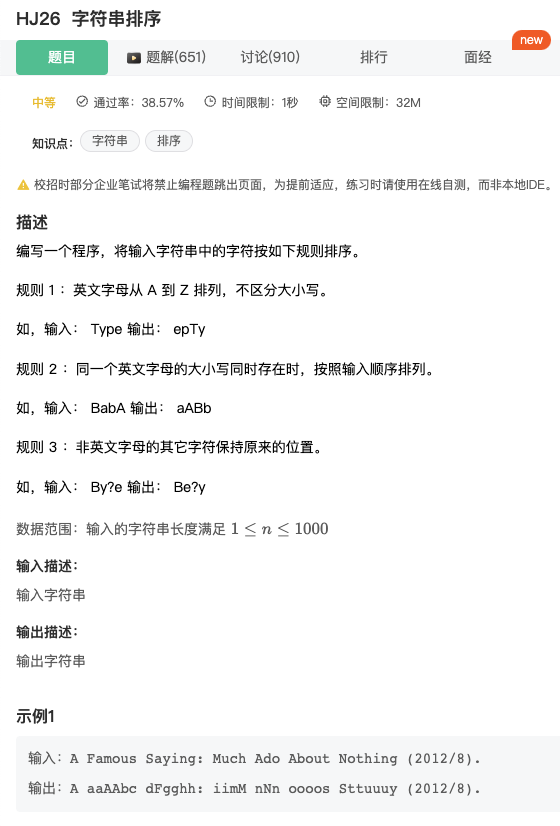
这题难度要大很多,关键是构建一个vector,先按字母的顺序存放字母。然后当遇到字母的时候,再次从vector中拿出来(对于同字母的大小写,按输入的顺序进行放入,也按输入的顺序进行取出)。注意通过处理原输入,可以避免处理其他不变的情况
字符串:坐标移动
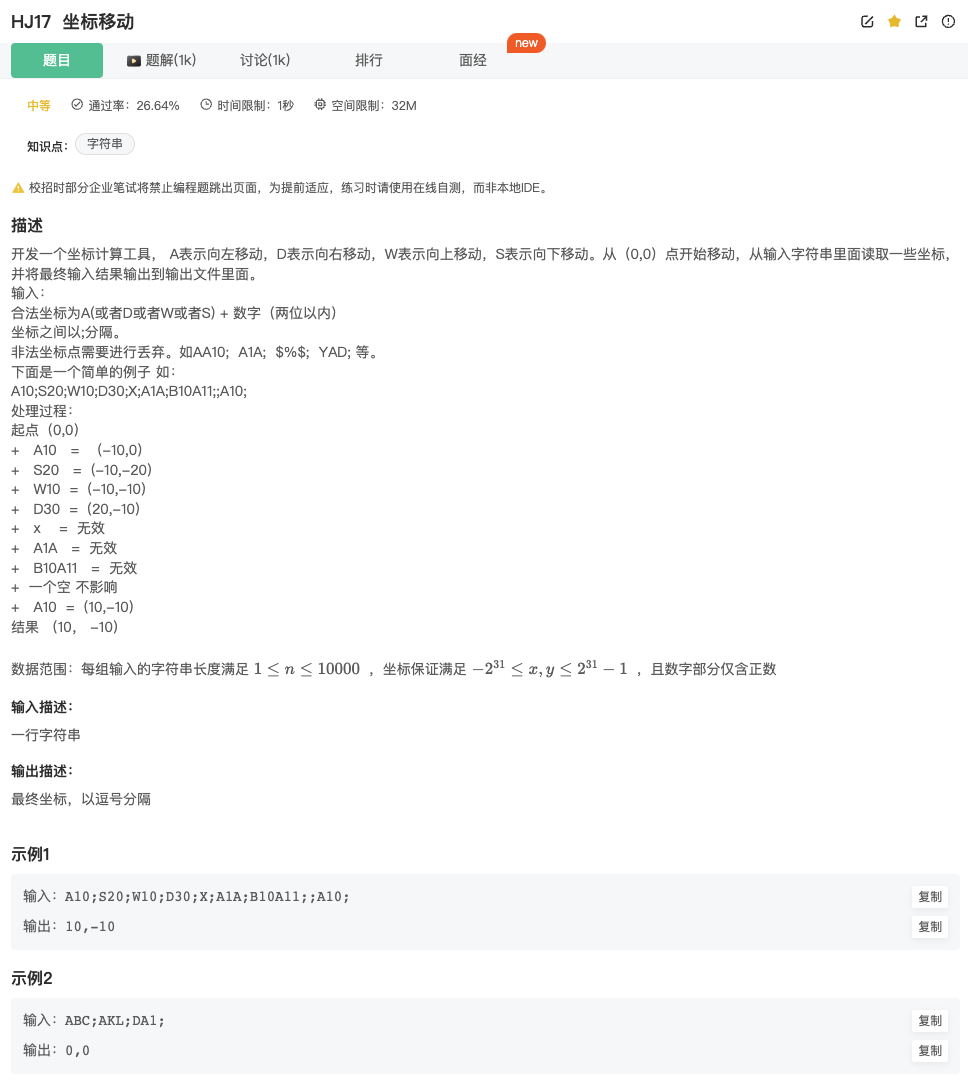
Click to expand the code
密码验证合格程序
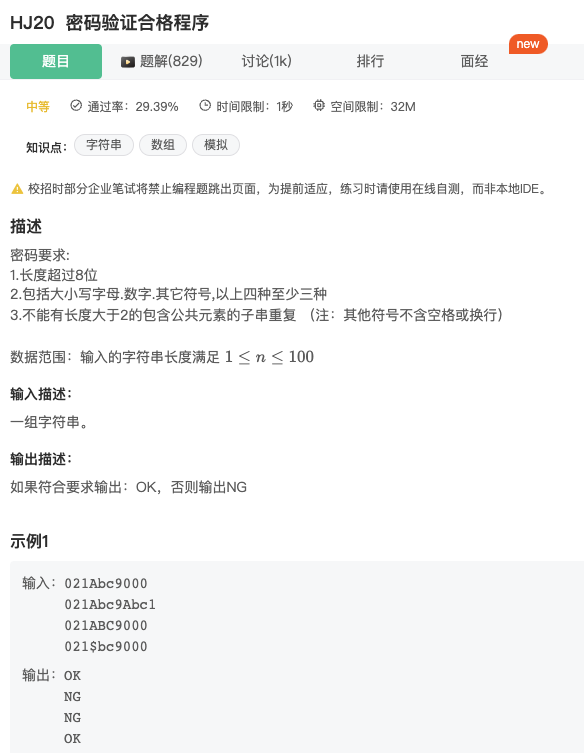
Click to expand the code
删除字符串中出现次数最少的字符
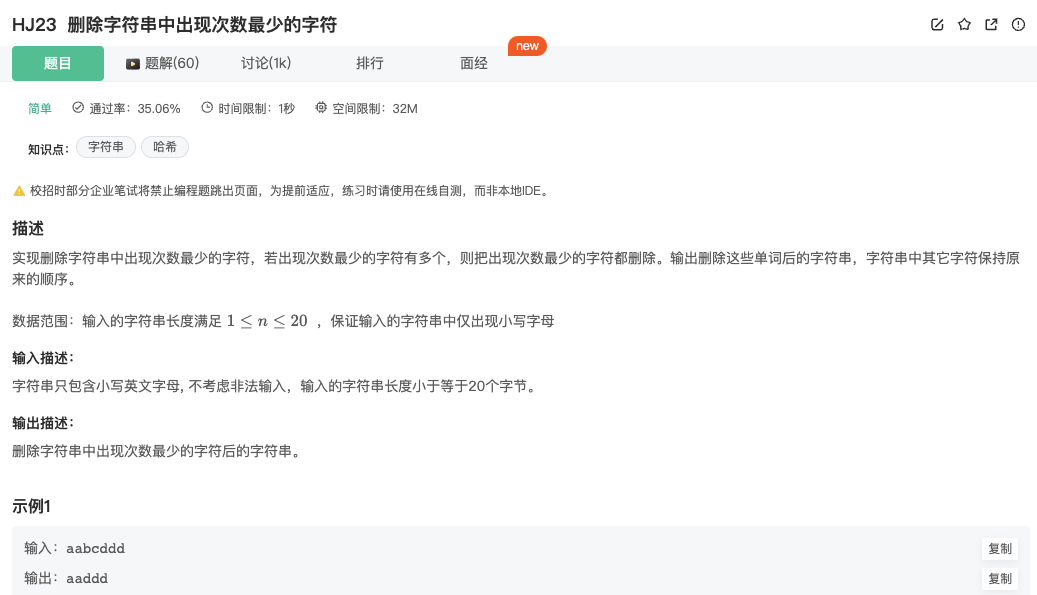
Click to expand the code
查找兄弟单词
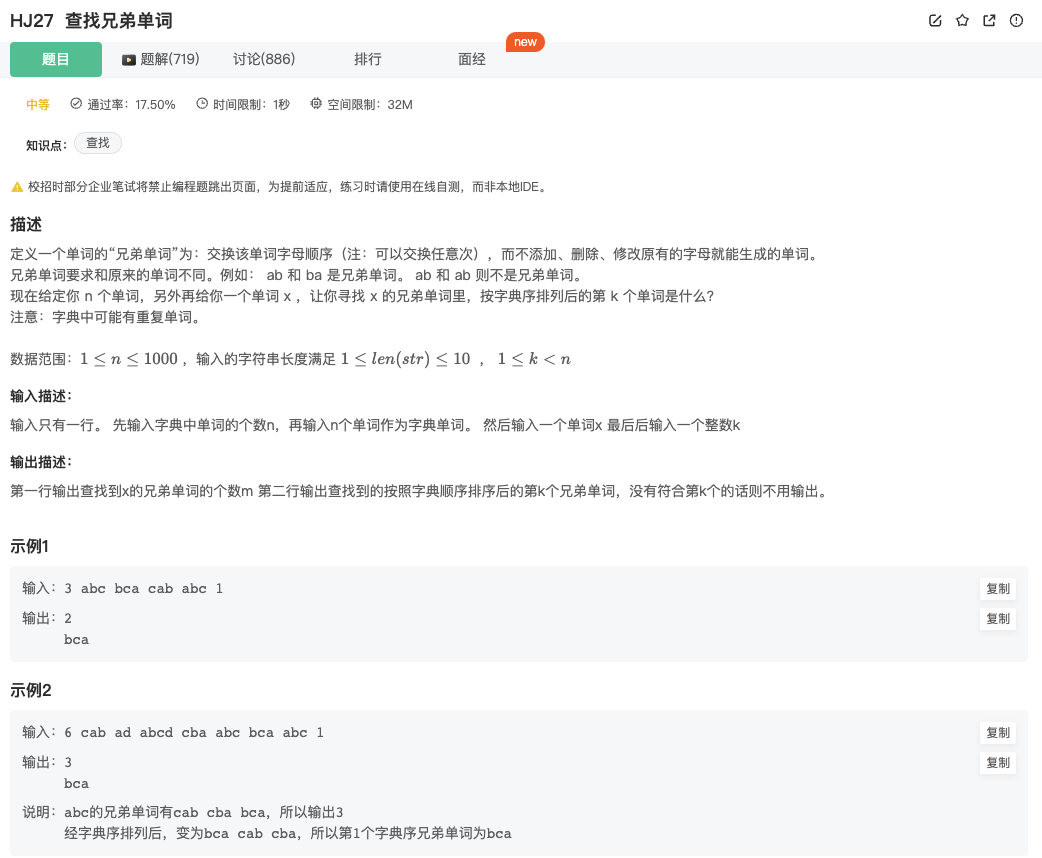
Click to expand the code。时间复杂度:O(nlogm),其中n为单词总数,m为最大单词的长度。logm为排序算法的复杂度。空间复杂度是O(N)注意最后一个单词才是目标,审题最重要!!!
字符串加解密
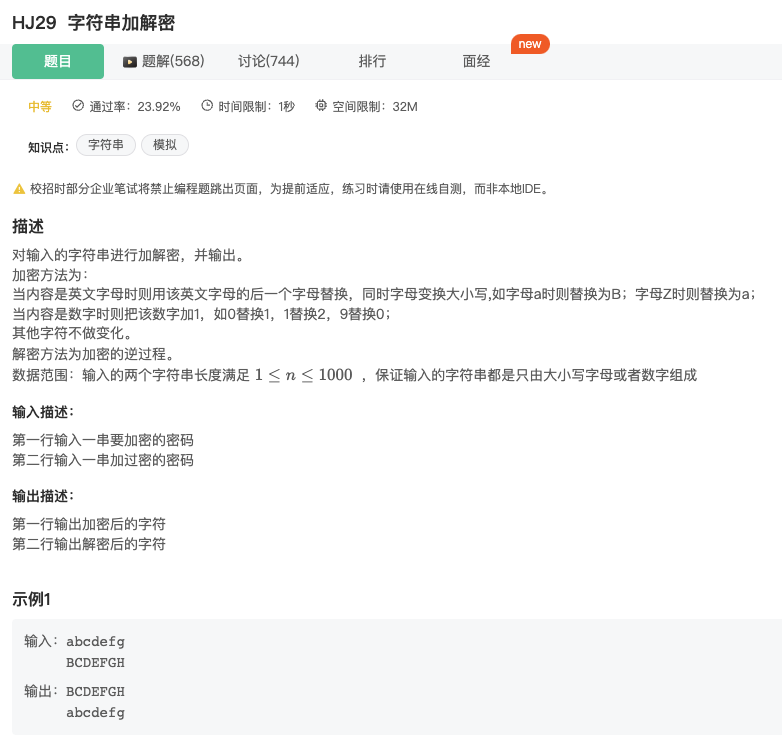
Click to expand the code。注意循环中用else if不然就会一直处理,一直叠加。
字符串反转
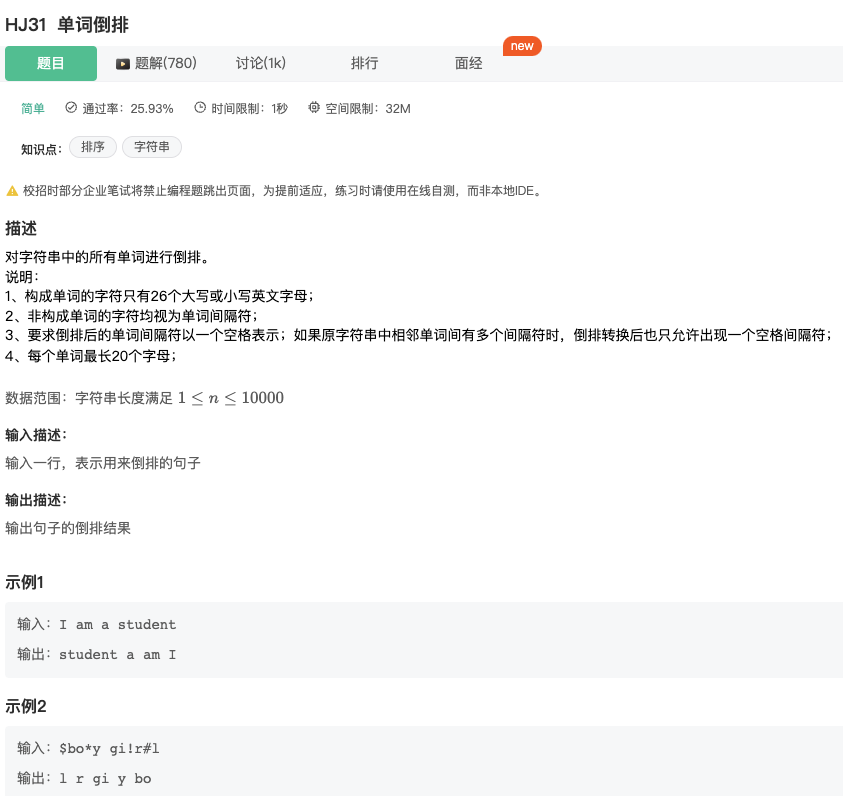
Click to expand the code
字符串相乘
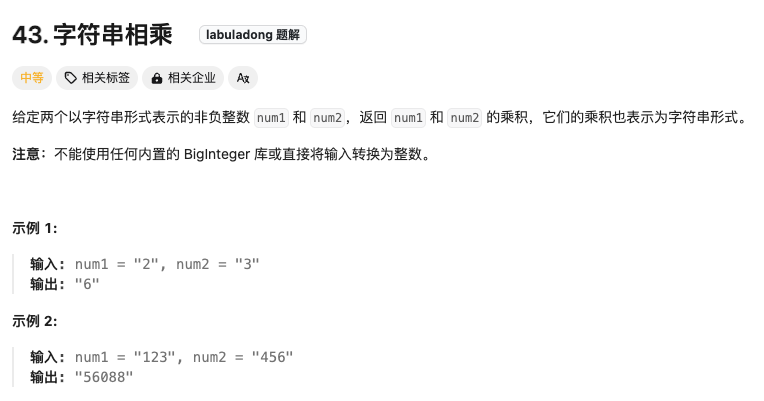
这个解法相对比较直接,就是把乘法的计算过程跟加的过程用代码实现了~
也可以采用下图所示的双指针法的思路解题。时间复杂度为O(n*m),空间复杂度为O(n+m)
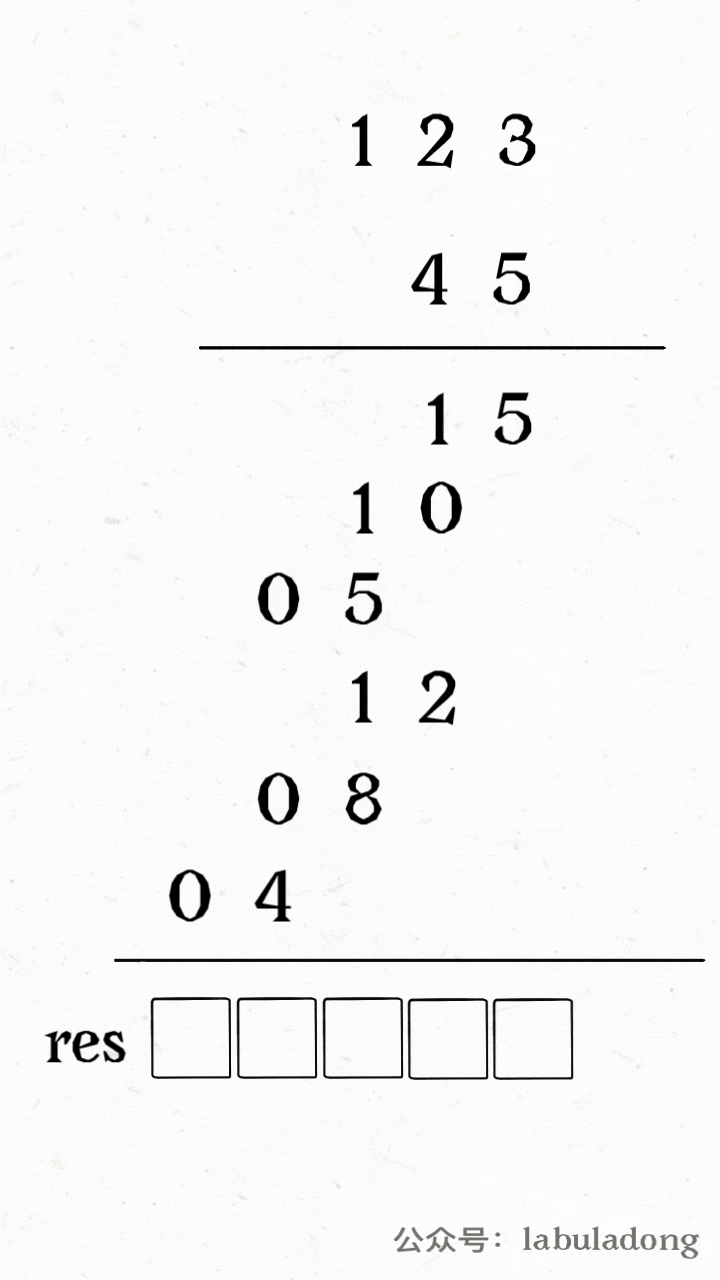
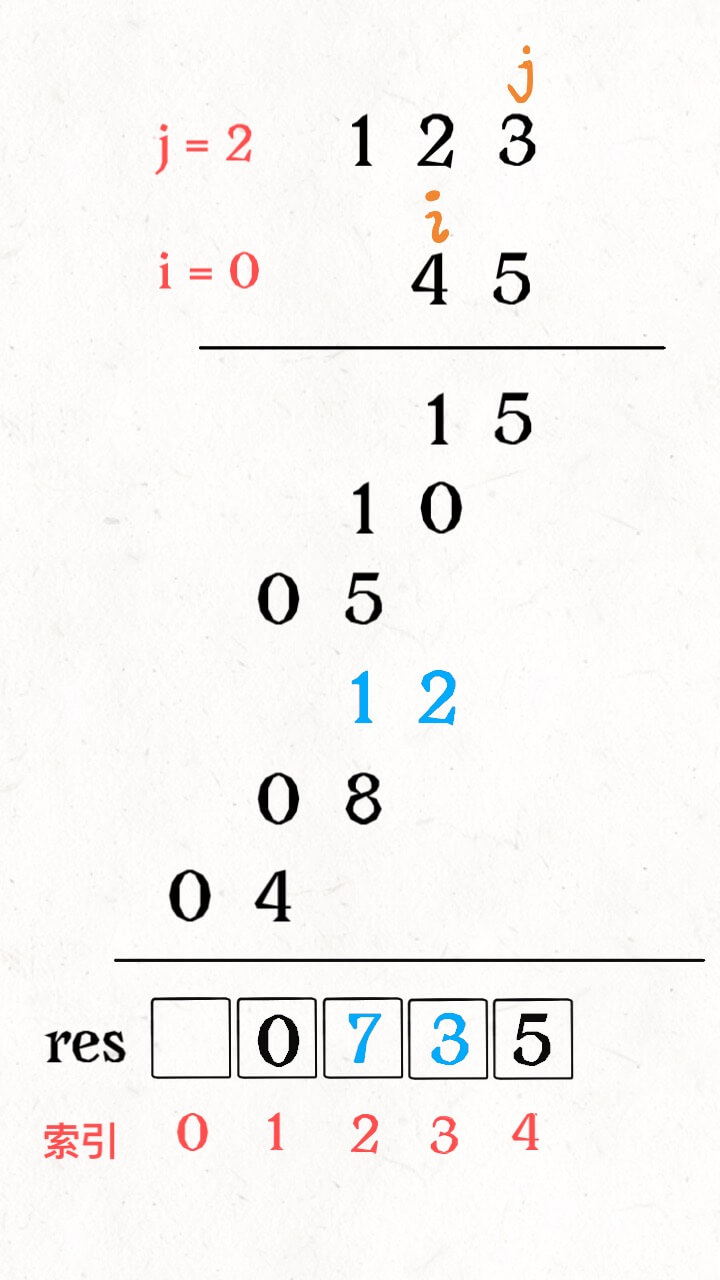
用python的eval函数求解就无敌简单😱
python求解字符串的四则运算
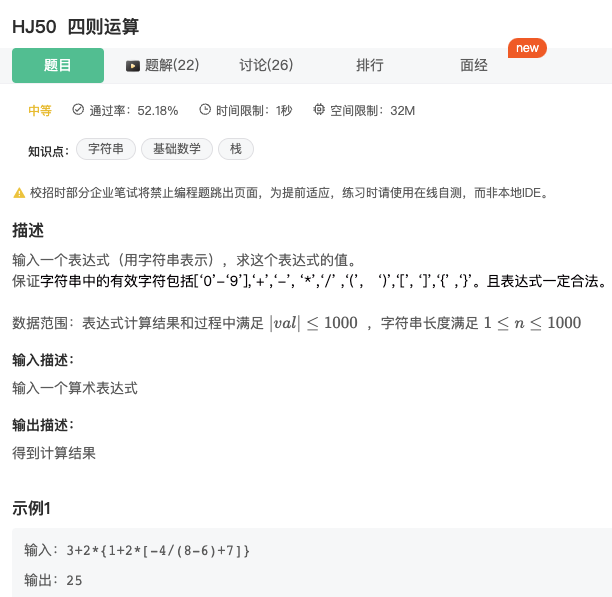
此题如果用cpp去解的话有点繁琐,为此改为用python,几句代码即可!
同理,上面字符串相乘也是可以类似的用
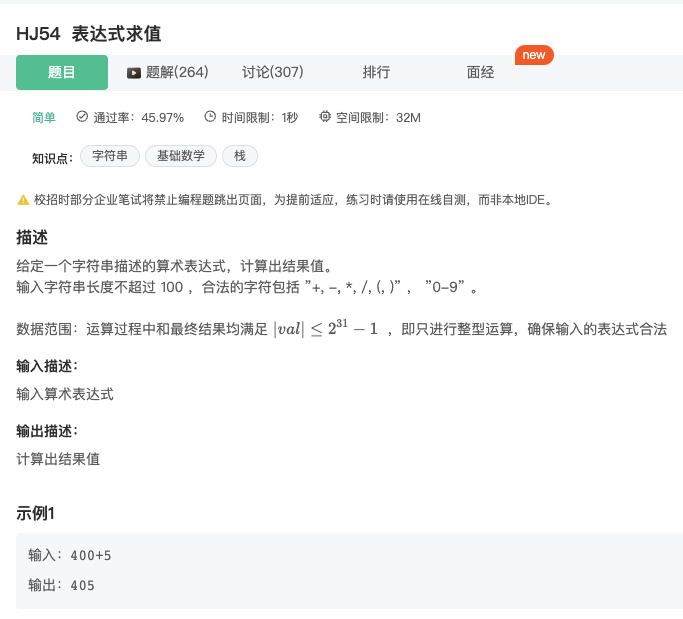
用python解题,三行代码即可!
高精度整数加法
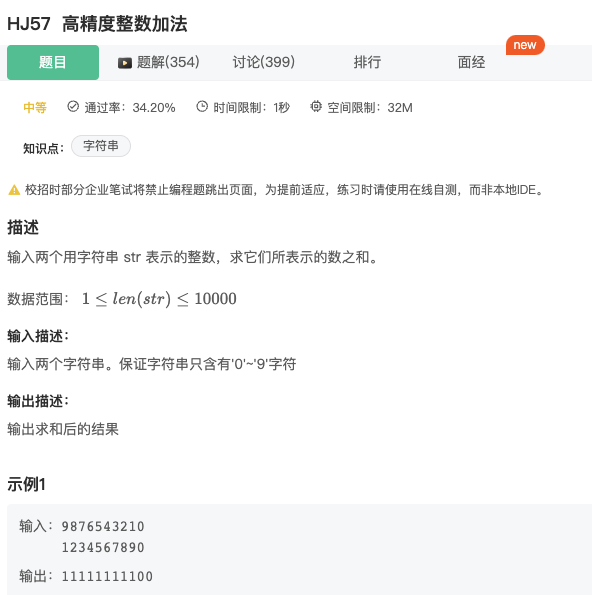
最简单就是采用python解题~
此处也给出cpp的解法,处理好进位以及字符串的反转即可~
蛇形矩阵
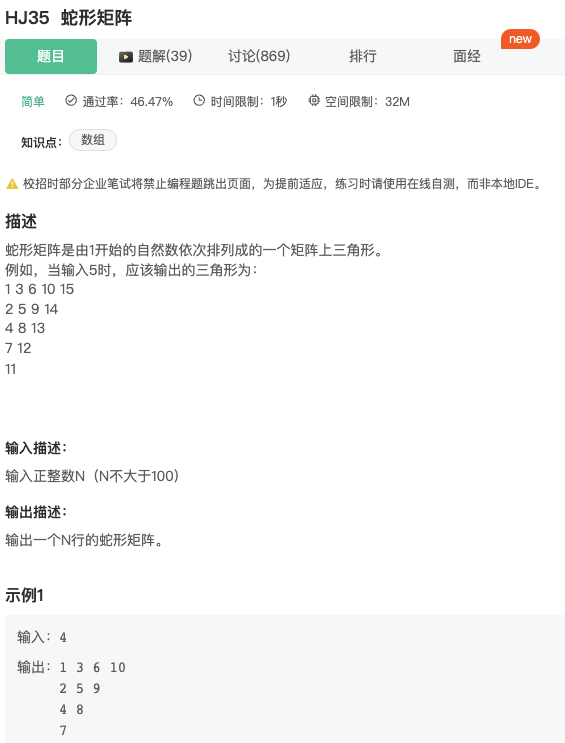
Click to expand the code.关键是如何填矩阵的思路~
挑7
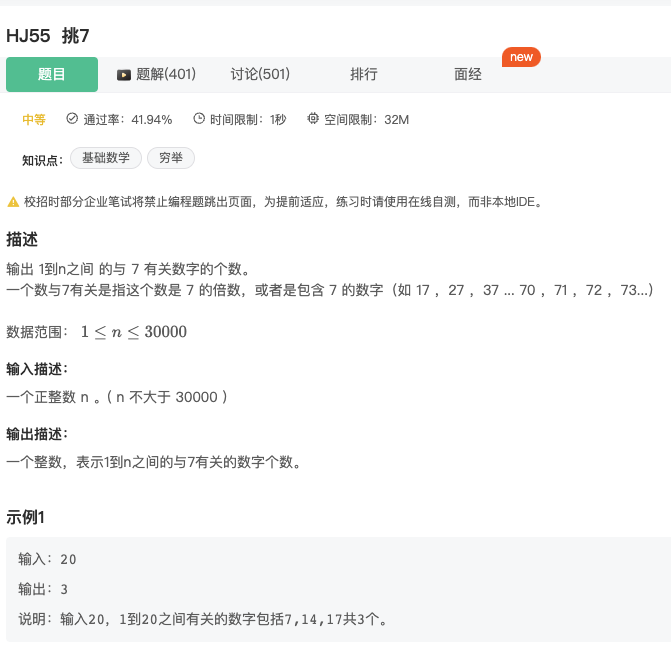
Click to expand the code.
查找两个字符串a,b中的最长公共子串
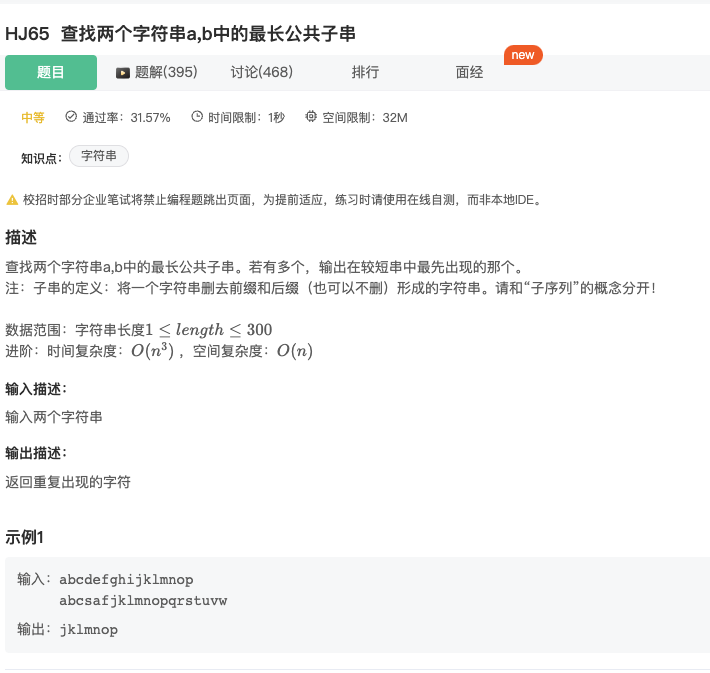
Click to expand the code.关键点是字符串中找子字符串的用法str_2.find(temp_str)!=string::npos
成绩排序
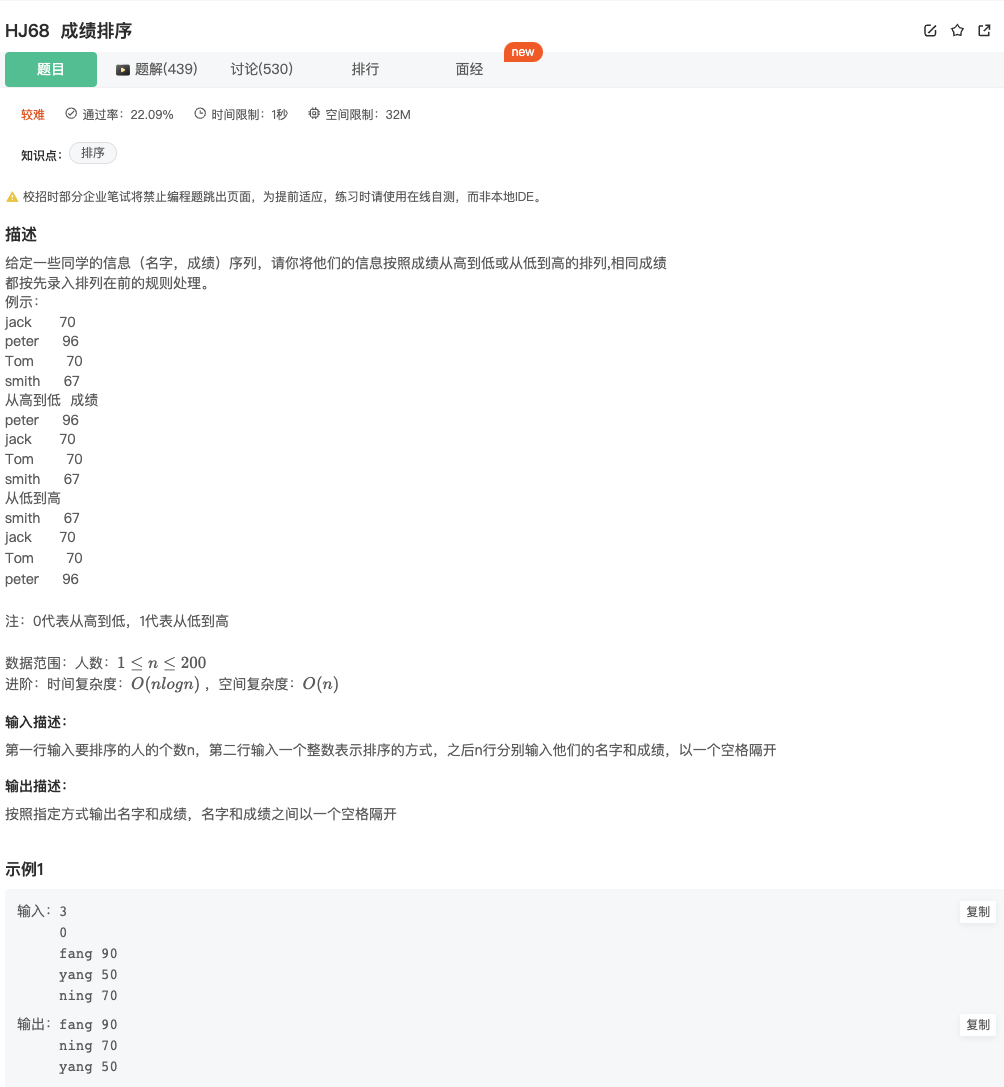
stable_sort 和 sort的区别在于 前者作排序可以使原来的"相同"的值在序列中的相对位置不变
在字符串中找出连续最长的数字串
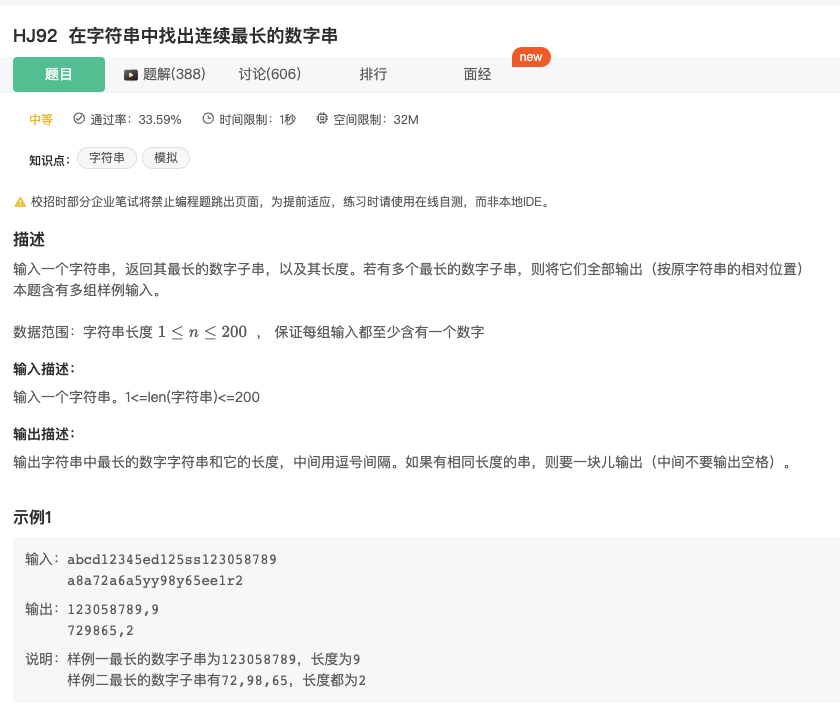
Click to expand the code
自守数
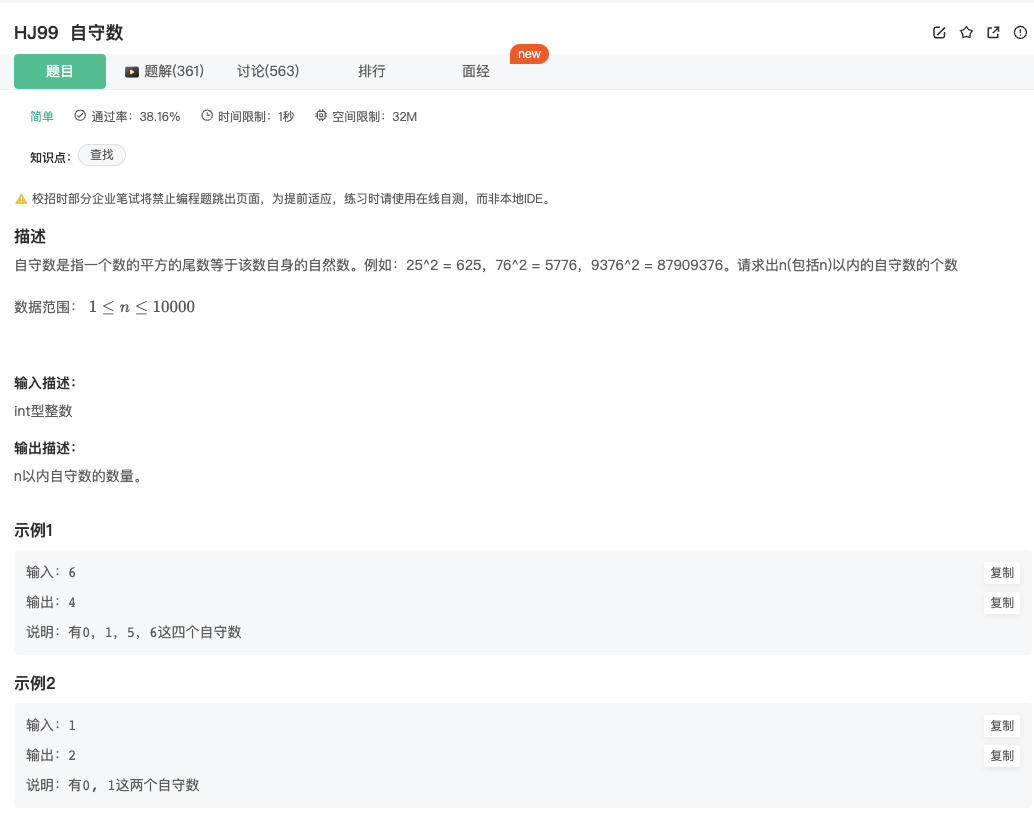
Click to expand the code